Introduction To Cameras
Today I will talk about Cameras.
What are Cameras? Cameras are the player’s eyes, it’s for them, that the player can has the experience, but this concept is very short, for a thing that is present in all games, so it’s not enough.
I cannot talk with this mode, so I will talk using a detailed mode, explaining uses, what is possible to do, how to work, etc.
Some camera uses in games:
– Create cutscenes.
– Create short presentations (for to present an environment or an enemy).
– Character’s Eyes.
– Camera’s Effects.
– Emphasize something (usually an item or goal).
So, now that I said a little about theory, let’s go to talk about the practice.
Unity gives us some options on camera components to configure some things, as you can see in the photo:
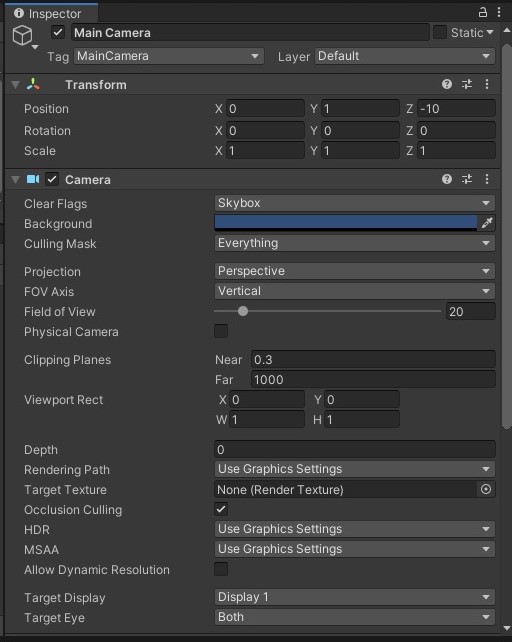
As you can see, We have many options to configure, I won’t talk about all, because otherwise the article will be too long, but I will talk about some properties such as: Field Of View (used in our practice example), Projection and Target Texture, so Let’s go.
- Field Of View – Change the Camera zoom, the higher the value the smaller the zoom, and the lower the value the greater the zoom.
- Projection – Change the visibility between 2D and 3D.
- Target Texture – Used with a render texture. A example of this use, is for to insert camera view in UI
Here is a link for Unity Documentation, where all the properties of the camera are explained: https://docs.unity3d.com/ScriptReference/Camera.html
But this is not all, it is possible to make more things, for example: it is possible to animate in camera GameObject, camera’s position, rotation, and scale (but scale doesn’t change anything in visibility).
Here is a photo:
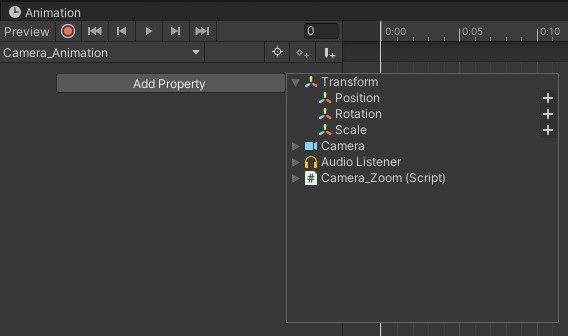
Also is possible to animate properties on Camera Component, we can to animate, for example: Allow MSAA, Depth, Enabled, Far clip plane, and others. I will insert photos of this window below.
Here is our first photo:
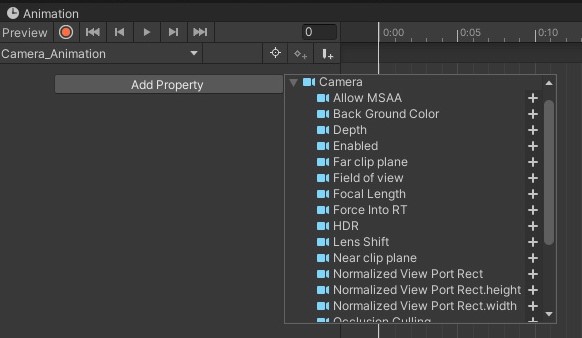
And here is our second Photo:
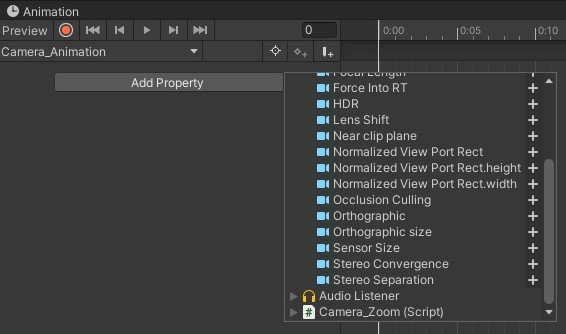
But this is not all, We can also create codes for work with our camera, and in this case, the limit is your knowledge.
For this article I will to make a Camera’s zoom (typically used in FPS Games) using Mouse’s right button, my camera zoom script will to have 3 Zoom options, that are:
– Default Zoom
– Medium Zoom
– Close Zoom
So let’s go to create our code.
Firstly, We need some variables, that are: an integer named _Zoom_Counter (for to count our mouse clicks), an integer named _Default_Zoom_Field_Of_View, an integer named _Medium_Zoom_Field_Of_View, an integer named _Close_Zoom_Field_Of_View, and for last, a Camera named _This_Cam, so this is our class:
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Camera_Zoom : MonoBehaviour { Camera _This_Cam; private int _Zoom_Counter; private float _Default_Zoom_Field_Of_View; private float _Medium_Zoom_Field_Of_View; private float _Close_Zoom_Field_Of_View; }
Now I will to define values for our variables in Start method, so here is our class:
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Camera_Zoom : MonoBehaviour { Camera _This_Cam; private int _Zoom_Counter; private float _Default_Zoom_Field_Of_View; private float _Medium_Zoom_Field_Of_View; private float _Close_Zoom_Field_Of_View; void Start() { _This_Cam = GetComponent<Camera>(); _Default_Zoom_Field_Of_View = 60; _Medium_Zoom_Field_Of_View = 20; _Close_Zoom_Field_Of_View = 3.3f; } }
Very well, now with our variables ready, I will to make in Update method a system input for to execute our zoom, when Right Mouse’s has been pressed, so here is our class:
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Camera_Zoom : MonoBehaviour { Camera _This_Cam; private int _Zoom_Counter; private float _Default_Zoom_Field_Of_View; private float _Medium_Zoom_Field_Of_View; private float _Close_Zoom_Field_Of_View; void Start() { _This_Cam = GetComponent<Camera>(); _Default_Zoom_Field_Of_View = 60; _Medium_Zoom_Field_Of_View = 20; _Close_Zoom_Field_Of_View = 3.3f; } void Update() { if (Input.GetKeyDown(KeyCode.Mouse1)) Check_Zoom(); } }
What is KeyCode.Mouse1? KeyCode.Mouse1 is responsible for to capture interaction with Right Mouse’s button.
So now We have, our input, but We don’t have our Check_Zoom method, for this method, I will firstly increase 1 in our variable named _Zoom_Counter that has value 0 currently, and so I will check _Zoom_Counter’s value with a switch, so here is our class with Check_Zoom method:
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Camera_Zoom : MonoBehaviour { Camera _This_Cam; private int _Zoom_Counter; private float _Default_Zoom_Field_Of_View; private float _Medium_Zoom_Field_Of_View; private float _Close_Zoom_Field_Of_View; void Start() { _This_Cam = GetComponent<Camera>(); _Default_Zoom_Field_Of_View = 60; _Medium_Zoom_Field_Of_View = 20; _Close_Zoom_Field_Of_View = 3.3f; } void Update() { if (Input.GetKeyDown(KeyCode.Mouse1)) Check_Zoom(); } void Check_Zoom() { _Zoom_Counter++; switch (_Zoom_Counter) { case 1: break; case 2: break; default: break; } } }
Now our script is taking shape, our method Check_Zoom has been created, but, We need to change value on our Camera’s field of view, so for a best practice I will make a method for each zoom, and after this, I will to call each method in your respective case, in switch instruction, so for to start, I will create Set_Default_Zoom_method, so here is our class:
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Camera_Zoom : MonoBehaviour { Camera _This_Cam; private int _Zoom_Counter; private float _Default_Zoom_Field_Of_View; private float _Medium_Zoom_Field_Of_View; private float _Close_Zoom_Field_Of_View; void Start() { _This_Cam = GetComponent<Camera>(); _Default_Zoom_Field_Of_View = 60; _Medium_Zoom_Field_Of_View = 20; _Close_Zoom_Field_Of_View = 3.3f; } void Update() { if (Input.GetKeyDown(KeyCode.Mouse1)) Check_Zoom(); } void Check_Zoom() { _Zoom_Counter++; switch (_Zoom_Counter) { case 1: break; case 2: break; default: break; } } void Set_Default_Zoom() { _This_Cam.fieldOfView = _Default_Zoom_Field_Of_View; } }
Now We can have idea that as our class will to be, now I will to create next methods, for Medium Zoom and Close_Zoom, here is our class:
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Camera_Zoom : MonoBehaviour { Camera _This_Cam; private int _Zoom_Counter; private float _Default_Zoom_Field_Of_View; private float _Medium_Zoom_Field_Of_View; private float _Close_Zoom_Field_Of_View; void Start() { _This_Cam = GetComponent<Camera>(); _Default_Zoom_Field_Of_View = 60; _Medium_Zoom_Field_Of_View = 20; _Close_Zoom_Field_Of_View = 3.3f; } void Update() { if (Input.GetKeyDown(KeyCode.Mouse1)) Check_Zoom(); } void Check_Zoom() { _Zoom_Counter++; switch (_Zoom_Counter) { case 1: break; case 2: break; default: break; } } void Set_Default_Zoom() { _This_Cam.fieldOfView = _Default_Zoom_Field_Of_View; } void Set_Medium_Zoom() { _This_Cam.fieldOfView = _Medium_Zoom_Field_Of_View; } void Set_Close_Zoom() { _This_Cam.fieldOfView = _Close_Zoom_Field_Of_View; } }
So with our methods created, We only need to call in our Check_Zoom_Method, but We also need to finish configuring our method, now I will make this.
In case 1, We will to use Set_Medium_Zoom
In case 2, We will to use Close_Zoom
In Default, we will set _Zoom_Counter as 0, and We will call, Set_Default_Zoom.
Lastly, We will call Set_Default_Zoom in Start method, for the case, the camera’s settings are different from our code.
So here is our finished class:
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Camera_Zoom : MonoBehaviour { Camera _This_Cam; private int _Zoom_Counter; private float _Default_Zoom_Field_Of_View; private float _Medium_Zoom_Field_Of_View; private float _Close_Zoom_Field_Of_View; void Start() { _This_Cam = GetComponent<Camera>(); _Default_Zoom_Field_Of_View = 60; _Medium_Zoom_Field_Of_View = 20; _Close_Zoom_Field_Of_View = 3.3f; Set_Default_Zoom(); } void Update() { if (Input.GetKeyDown(KeyCode.Mouse1)) Check_Zoom(); } void Check_Zoom() { _Zoom_Counter++; switch (_Zoom_Counter) { case 1: Set_Medium_Zoom(); break; case 2: Set_Close_Zoom(); break; default: _Zoom_Counter = 0; Set_Default_Zoom(); break; } } void Set_Default_Zoom() { _This_Cam.fieldOfView = _Default_Zoom_Field_Of_View; } void Set_Medium_Zoom() { _This_Cam.fieldOfView = _Medium_Zoom_Field_Of_View; } void Set_Close_Zoom() { _This_Cam.fieldOfView = _Close_Zoom_Field_Of_View; } }
So for this article is this.
I talked about cameras, and I made a simple example of something using Camera.
This project is in my github https://github.com/MauroJrDeveloper/Camera_Project
Follow-me in Instagram for stay knowing when I create new posts @mauro_developer
You can also leave your feedback, and your suggestion, I will read all, and I will answer for you.
I would like to give a special thanks to the reader Anderson Monte who suggested that I do an article about cameras.
Thank you for reading.